|
NOTE:This is a read only archive of threads posted to the FreeRTOS support forum. Use these archive pages to search previous posts. New forum support threads can be started at the FreeRTOS forums.
FreeRTOS Support Archive
The FreeRTOS support forum can be used for active support both from Amazon Web Services and the community. In return for using our software for free, we request you play fair and do your bit to help others! Sign up for an account and receive notifications of new support topics then help where you can.
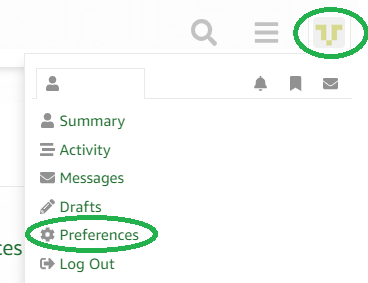
This is a read only archive of threads posted to the FreeRTOS support forum. Use these archive pages to search previous posts. New forum support threads can be started at the FreeRTOS forums.
[FreeRTOS Home]
[Live FreeRTOS Forum]
[FAQ]
[Archive Top]
[October 2014 Threads]
task creation problemPosted by tomirtos on October 9, 2014 Hi everyone!
I had a hard time to get to this point in my project, and I’ve been halted again.
My simple code compiles fine, yet when I upload it to my microcontroller it won’t do anything it is supposed to do.
I am using a dsPIC33FJ128MC802 microcontroller with MPLABx xc16 compiler and microstick2 test board. I managed to configure one of the PIC24 sample project to my dsPIC. Also I can blink leds on their own and I included my own UART functions that send characters to the console and that also works fine under the RTOS.
The problem starts when I want to start making tasks. I have no idea how to even start looking for the source of the error. Any help would be really appreciated!
So here is my line of code:
void send_task(void *p)
{
while (1)
{
unsigned char data='k';
UART1PutChar(data);
}
}
int main (void)
{
pll_setup(); // makes 40 MIPS
init_UART1(); // Initialize UART1 for 9600,8,N,1 TX/RX
prvSetupHardware();
//make task
xTaskCreate(send_task, "send_task", 4096, 0, 1, 0);
vTaskStartScheduler();
//UART TEST, this works by itself
/* while (1)
{
char data=’k’;
UART1PutChar(data);
}*/
return 0;
}
task creation problemPosted by rtel on October 9, 2014 I’ve read this twice so am pretty sure you make no mention of what the problem actually is, so I’m left at guessing….
…and my guess would be that the task is not even being created because you have requested a massive stack size – which by itself would consume half of the RAM available on that part.
Have you:
1) Checked the return value of xTaskCreate().
2) Stepped into the xTaskCreate() function in the debugger to actually see why it is failing, if indeed it is failing.
3) Defined a malloc failed hook to see when you run out of RAM?
Regards.
task creation problemPosted by tomirtos on October 9, 2014 Sorry you are right, I’ll try and explain what it should do:
So I should be able to see from my serial port monitor the letter “k” beeing sent. If I am not using my uart function in a task it does just that, but if I put it in a task, nothing appears on the serial monitor. In my compiler the xTaskCreate line is underlined in red, meaning it is not recognised. But as I said the code compiles without errors.
If I set a breakpoint at xTaskCreate and save it to a variable the variable will be equal to 0.
If I enter debugging mode, the program halts and I have to manually press the green continue button. I see these messages on the debugger console:
No source code lines were found at current PC 0x3d0
User program stopped
User program running
No source code lines were found at current PC 0x0
User program stopped
User program running
No source code lines were found at current PC 0x3d0
User program stopped
task creation problemPosted by rtel on October 9, 2014
If I set a breakpoint at xTaskCreate and save it to a variable the
variable will be equal to 0.
Does that mean if you save the return value of xTaskCreate() to a
variable, then the return value is 0? In which case xTaskCreate() is
failing probably for the reason stated in my previous reply.
Regards.
task creation problemPosted by tomirtos on October 9, 2014 Thank you so far for the help.
So I’ve reduced the stack to 512. If I step into the xTaskCreate function during debugging I can see it returns with the xReturn variable beeing 1. So I guess that means it is beeing created. Unfortunately still nothing appears on my console.
task creation problemPosted by rtel on October 9, 2014 …and you are writing to the UART from inside the task? The code you
pasted shows the UART being used after vTaskStartScheduler() is called.
If all is well vTaskStartScheduler() will not return, so any code
after that will not execute.
Regards,
Richard.
task creation problemPosted by tomirtos on October 9, 2014 No, i was not writing the UART after the vTaskStartScheduler. It is commented out. I left it in there only to show that that line of code was working on its own without beeing in a task. So my UART functions are working fine and the problem is with them beeing in a task.
I am writing to the UART in the send_task function.
task creation problemPosted by rtel on October 9, 2014 If you put a break point inside the task – does it get hit (showing the
task executes)? Another way of seeing if the task is executing is to
declare a global volatile variable, then increment the variable on each
loop of the task.
If the task is being executed, can you post the code for UART1PutChar()
– or at least say whether it is using interrupt or not.
Regards.
task creation problemPosted by tomirtos on October 9, 2014 I tried putting a break point inside sender_task function. It seems my program never reaches that break point. This is probably not a good sign..
This is the code for the UART1PutChar: It utilizes no interrupts.
void UART1PutChar(char Ch)
{
//transmit ONLY if TX buffer is empty
while(U1STAbits.UTXBF == 1);
U1TXREG = Ch;
}
task creation problemPosted by rtel on October 9, 2014 UART1PutChar() is as simple as can be, so can’t be the cause of the
problem – but it seems the task is not actually running in the first
place. The next thing to check is whether vTaskStartScheduler()
returns. If it returns it would again be a symptom of there not being
enough heap space. Did you define a malloc failed hook so you would
know immediately if this was the case?
Regards.
task creation problemPosted by tomirtos on October 9, 2014 Sorry I had to leave and couldn’t continue working on my project. I’m afraid I don’t think I know how to define a malloc failed hook, or understand what that really means. I will try out the global volatile variable solution.
task creation problemPosted by edwards3 on October 9, 2014 Set configUSEMALLOCFAILED_HOOK to 1 in FreeRTOSConfig.h, then add a function called vApplicationMallocFailedHook() into your code. If a call to pvPortMalloc in the xTaskCreate() function fails it will call vApplicationMallocFailedHook() so if you implement it like this
void vApplicationMallocFailedHook(void){
portDISABLE_INTERRUPTS();
while(1);
}
you will end up in the while(1) if it is called.
See http://www.freertos.org/a00016.html
task creation problemPosted by tomirtos on October 10, 2014 Thank you for the explanation! I will try it out now, but first I did the global variables:
I declared three variables. ‘A’ is placed inside the send_task, and it never gets incremented, so the program never gets there.
B is between the taskcreate and taskscheduler, it only gets incremented once and C is after the task scheduler, which never gets incremented. So this means that the program is in vTaskStartScheduler.
I also get this message when in debugger mode in call stack view:
No source code lines were found at current PC 0x29e . When I hit continue button it stops again with the same message except now PC is at 0x00. And if I keep hitting continue it keeps on toggling between these to addresses. Maybe this is the source of the problem aswell.
This is my source code for global variables.
volatile static int A=0;
volatile static int B=0;
volatile static int C=0;
void send_task(void *p)
{
while (1)
{
A=A+1; //never gets incremented
unsigned char data=’k’;
UART1PutChar(data);
vTaskDelay(500);
}
}
int main (void)
{
pll_setup(); // set internal oscillator to 80MHz-->40MIPS FCY=Fosc/2
init_UART1(); // UART enable
xTaskCreate(send task, “sendtask”, 512, NULL, 1, NULL);
B=B+1; // only gets incremented once
vTaskStartScheduler();
C=C+1; //never gets incremented
return 0;
}
task creation problemPosted by tomirtos on October 10, 2014 I tried out the malloc failed hook function. Implemented as you suggested. One thing was strange that I didn’t have a predefined configUSEMALLOCFAILED_HOOK in my FreeRTOSConfig.h file, but I created one.
So when I put a break point in vApplicationMallocFailedHook function the compiler never hits it and also I never enter the while(1) loop. So I don’t think its a memory allocation problem.
task creation problemPosted by davedoors on October 10, 2014
No source code lines were found at current PC 0x29e
I think you need to find the reason for that first. It sounds like the code is running up to a point, then simply crashing. Could be a linker script problem. You know the code runs up to B=B+1, so step through the code from there until you find the code line that jumps into the weeds.
task creation problemPosted by tomirtos on October 10, 2014 Thank you so far for the help. I tried what you suggested and I think this is where I lose where the program is going:
The program enters xPortStartScheduler. It reaches the break point at portRESTORE_CONTEXT();
If I set the next breakpoint to be at asm volatile (“return”); it will never get hit.
If I set the break point at return pdTRUE it wont get hit either.
I tried setting breakpoints where portRESTORE_CONTEXT(); is defined but for some reason I can’t make any breakpoints there.
BaseType_t xPortStartScheduler( void )
{
/* Setup a timer for the tick ISR. */
vApplicationSetupTickTimerInterrupt();
/* Restore the context of the first task to run. */
portRESTORE_CONTEXT();
/* Simulate the end of the yield function. */
asm volatile ( "return" );
/* Should not reach here. */
return pdTRUE;
}
Copyright (C) Amazon Web Services, Inc. or its affiliates. All rights reserved.
|
|